In today’s rapidly evolving software development landscape, ensuring the quality of applications has become a critical aspect of delivering a seamless user experience. Quality Assurance (QA) plays a vital role in achieving this goal by implementing various testing methodologies.
Automation testing is one such methodology that has gained significant popularity due to its ability to streamline the testing process and improve efficiency. Among the numerous automation testing tools available, Selenium stands out as a powerful and versatile option.
When combined with Python, Selenium becomes an even more potent tool for automation testing. In this article, we will explore the best practices of using Selenium with Python for automation testing to achieve robust and reliable software applications.
Why Selenium and Python?
Before delving into the specifics of Selenium automation testing with Python, let’s understand why these two technologies are widely chosen by QA professionals. Selenium is an open-source web automation testing tool known for its flexibility and compatibility with multiple programming languages.
It supports various operating systems and web browsers, making it a versatile choice for testing web applications. Python, on the other hand, is a high-level, easy-to-learn programming language that is widely used for automation testing, data extraction, and other tasks. Python’s simple syntax and extensive library support make it an ideal companion for Selenium. The combination of Selenium and Python offers several advantages, including:
- Ease of Use: Python’s simplicity and readability make it easy for beginners to grasp the language and write automation scripts. The intuitive syntax, resembling English words, allows testers to quickly learn and start using Selenium WebDriver for web automation testing without facing steep learning curves.
- Extensive Community Support: Python has a large and vibrant community of developers who actively contribute to the development of libraries, frameworks, and resources specifically tailored for Selenium automation. This abundance of community support ensures that there are ample resources available to solve problems, find answers to questions, and access ready-to-use code snippets and examples.
- Wide Range of Libraries and Frameworks: Python boasts an extensive ecosystem of libraries and frameworks that can be integrated with Selenium WebDriver for enhanced automation testing. These libraries and frameworks provide additional functionalities, such as handling database connections, generating test reports, interacting with APIs, and more. With Python, testers can easily integrate other tools and technologies into their automation workflow, making it a powerful and customizable solution.
- Cross-Platform Compatibility: Selenium WebDriver with Python is compatible with various operating systems, including Windows, macOS, and Linux. This cross-platform compatibility allows testers to write automation scripts on one platform and seamlessly execute them on different operating systems without major modifications. This flexibility saves time and effort, as applications can be tested across different environments to ensure compatibility with diverse user setups.
- Integration Capabilities: Python’s versatility extends beyond Selenium WebDriver. It can be effortlessly integrated with other tools and technologies commonly used in the software development and testing landscape. Test management systems, continuous integration tools, logging frameworks, and reporting tools can be seamlessly integrated into the automation workflow, enabling testers to build a comprehensive and customized automation ecosystem tailored to their specific project requirements.
Selenium Automation Testing Tools
Selenium is not just a single tool but a collection of tools, each catering to different automation testing needs.
Let’s take a closer look at the Selenium automation testing tools that can be utilized with Python.
Selenium IDE (Integrated Development Environment)
Selenium IDE is an easy-to-use interface that records user interactions to build automated test scripts. It is a Firefox or Chrome plugin primarily used as a prototyping tool to speed up the creation of automation scripts. Although Selenium IDE was discontinued in August 2017, it has recently been reimagined and relaunched with several advancements. These advancements include reusability of test scripts, debugging capabilities, the Selenium side runner, provision for control flow statements, improved locator functionality, and more.
To install Selenium IDE, follow these steps:
- Open the Firefox browser.
- Click on the menu in the top-right corner and select “Add-ons” from the drop-down menu.
- Click on “Find more add-ons” and type “Selenium IDE” in the search bar.
- Click on “Add to Firefox” to install Selenium IDE.
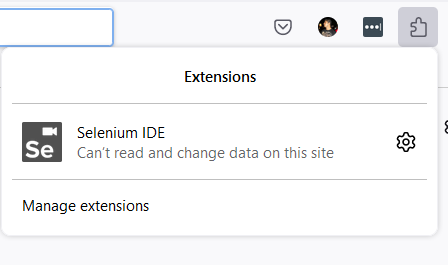
Once installed, the Selenium IDE icon will appear in the top-right corner of the browser. Clicking on it will display a welcome message, indicating that the IDE is ready for use.
Selenium Remote Control (RC)
Selenium Remote Control, also known as Selenium RC or Selenium 1, was developed to address a limitation of Selenium Core related to accessing elements of different domains.
Selenium RC is a server written in Java that allows writing application tests in various programming languages like Java, C#, Perl, PHP, Python, and more. The RC server acts as a client-configured HTTP proxy, tricking the browser into believing that Selenium Core and the web application being tested share the same origin.
Selenium RC is ideal for testing web applications across different browsers and platforms.
Selenium WebDriver
Selenium WebDriver, developed by Simon Stewart in 2006, offers a cross-platform testing framework that configures and controls browsers at the OS level. Unlike Selenium RC, WebDriver does not require a core engine and interacts natively with browser applications.
It supports multiple programming languages, including Python, Ruby, PHP, and Perl, making it a versatile choice for automation testing. Selenium WebDriver can also be integrated with frameworks like TestNG and JUnit for test management.
The architecture of Selenium WebDriver is simple and easy to understand. It consists of a Selenium test script, the JSON Wire Protocol for data transfer, browser drivers to establish a secure connection with browsers, and the browsers themselves.
This architecture allows for seamless communication between the test script and the browser, enabling comprehensive automation testing.
Getting Started with Selenium WebDriver and Python
To begin using Selenium WebDriver with Python, follow these steps:
- Download and Install Python: If Python is not already installed on your system, download and install the latest version of Python from the official website (https://www.python.org). Follow the installation instructions provided for your operating system.
- Install Selenium WebDriver: Once Python is installed, open the command prompt or terminal and install Selenium WebDriver using the pip package manager. Run the following command:
pip install selenium
This command will download and install the latest version of Selenium WebDriver.
- Choose a WebDriver: Selenium WebDriver requires a browser-specific driver to interact with the browser. Different browsers have their respective drivers, such as ChromeDriver for Google Chrome, GeckoDriver for Mozilla Firefox, and WebDriver for Microsoft Edge. Download the appropriate driver for the browser you intend to use for testing and ensure it is in your system’s PATH.
- Import Selenium WebDriver in Python: Open your preferred Python integrated development environment (IDE) or text editor and create a new Python script. Import the necessary modules from the Selenium library to establish a connection with the browser and automate test scenarios. For example, to automate testing with Chrome, use the following import statements:
from selenium import webdriver from selenium.webdriver.common.keys import Keys
These import statements allow you to work with the WebDriver and emulate keyboard key strokes for test scenarios.
- Create an Instance of the WebDriver: Use the appropriate WebDriver class to create an instance of the browser you want to automate. For example, to create an instance of Chrome WebDriver, use the following code:
driver = webdriver.Chrome('./chromedriver')
This code assumes that the ChromeDriver executable is located in the same directory as your Python script. Adjust the path accordingly if necessary.
- Load a Webpage: Use the
get()
method of the WebDriver instance to load a webpage. For example, to load the Python website, use the following code:
driver.get("https://www.python.org")
This code will navigate to the specified URL and load the webpage in the browser.
- Perform Interactions: Selenium WebDriver allows you to interact with elements on the webpage using various methods. For example, to search for an element by its name and enter a value, use the following code:
search_bar = driver.find_element_by_name("q") search_bar.clear() search_bar.send_keys("getting started with Python") search_bar.send_keys(Keys.RETURN)
This code finds the search bar element by its name, clears any existing value, enters the desired search query, and presses the Enter key to submit the search.
- Verify Results: After performing interactions, you can verify the results or extract information from the webpage. For example, to print the current URL, use the following code:
print(driver.current_url)
This code will display the current URL in the console.
- Close the WebDriver: Once the testing is complete, close the WebDriver instance to release system resources. Use the
close()
method as follows:
driver.close()
This code will close the browser window associated with the WebDriver instance.
Best Use Cases of Selenium WebDriver with Python
Selenium WebDriver with Python can be utilized for various automation testing scenarios. Let’s explore the top five best use cases where Selenium WebDriver and Python shine:
1. Web Application Testing
Selenium WebDriver with Python is best known for its ability to automate tests for web applications. From form submissions to data validation, WebDriver allows testers to simulate real user interactions and ensure the functionality and reliability of web applications across different browsers and platforms.
2. Cross-Browser Testing
Testing web applications across multiple browsers is essential to ensure consistent performance and user experience. Selenium WebDriver with Python enables testers to write tests once and execute them on various browsers, such as Chrome, Firefox, Edge, Safari, and more. This cross-browser testing ensures compatibility and identifies any browser-specific issues.
3. Regression Testing
Regression testing involves retesting previously tested functionality to ensure that recent changes or bug fixes have not introduced new issues. Selenium WebDriver with Python simplifies regression testing by automating repetitive test scenarios, allowing testers to focus on critical areas and reducing the overall testing time.
4. Data-Driven Testing
Data-driven testing involves executing a single test scenario with multiple sets of test data. Selenium WebDriver with Python integrates well with Python’s data manipulation capabilities, allowing testers to automate data-driven testing. By reading test data from external sources such as spreadsheets or databases, testers can validate application behavior under different data conditions.
5. Continuous Integration and Delivery (CI/CD) Pipeline Testing
In modern software development practices, integrating testing into the CI/CD pipeline is crucial for ensuring the quality and stability of applications. Selenium WebDriver with Python can be seamlessly integrated into the CI/CD pipeline, allowing automated tests to be triggered automatically with each code commit. This integration ensures that any issues are identified early in the development process, enabling rapid feedback and faster delivery of high-quality software.
Example: Selenium WebDriver Test with Python and GitLab CI/CD Pipeline
Let’s walk through an example of how to use Selenium WebDriver with Python in a GitLab CI/CD pipeline to automate testing.
Prerequisites
- Ensure that GitLab CI/CD is set up and configured for your project.
- Create a
.gitlab-ci.yml
file in the root of your project repository. - Ensure that ChromeDriver is installed and available in the CI/CD runner environment.
.gitlab-ci.yml
Configuration
The following is an example .gitlab-ci.yml
configuration file for running Selenium WebDriver tests with Python:
stages: - test test: image: python:3.9 stage: test script: - pip install selenium - apt-get update && apt-get install -y chromium-driver - python -m unittest tests.py
This configuration sets up a test stage that runs on a Python 3.9 image. It installs the Selenium library, installs the ChromeDriver package, and executes the tests.py
script using the python -m unittest
command.
Sample Test Script (tests.py
)
Here is a sample test script written in Python using Selenium WebDriver:
import unittest from selenium import webdriver from selenium.webdriver.common.keys import Keys class WebApplicationTests(unittest.TestCase): def setUp(self): self.driver = webdriver.Chrome() def test_search_functionality(self): driver = self.driver driver.get("https://www.example.com") search_bar = driver.find_element_by_name("q") search_bar.clear() search_bar.send_keys("example search") search_bar.send_keys(Keys.RETURN) self.assertIn("Search Results", driver.title) def tearDown(self): self.driver.close() if __name__ == "__main__": unittest.main()
This test script sets up a test case for the search functionality of a web application. It uses the Chrome WebDriver, navigates to the application’s homepage, enters a search query, and verifies that the search results page is displayed.
Running the Test in GitLab CI/CD Pipeline
When you push your changes to the GitLab repository, the CI/CD pipeline will be triggered automatically. The pipeline will install the necessary dependencies, execute the test script using Selenium WebDriver with Python, and provide the test results and feedback.
By incorporating Selenium WebDriver tests into your CI/CD pipeline, you can ensure that any changes to your application are thoroughly tested and validated, enabling faster and more reliable software delivery.
Conclusion
Quality Assurance is a crucial aspect of software development, and automation testing plays a significant role in ensuring the quality and reliability of applications. Selenium WebDriver, when combined with Python, offers a powerful and versatile solution for automation testing. The ease of use, extensive community support, wide range of libraries and frameworks, cross-platform compatibility, and integration capabilities make Selenium WebDriver with Python an ideal choice for QA professionals.
By harnessing the power of Selenium WebDriver with Python, QA professionals can enhance their automation testing efforts and deliver high-quality software applications that meet the expectations of end users, ensuring a seamless and satisfying user experience.
Happy testing!