Are you a web developer seeking to take your skills to the next level? Look no further! In this article, we will explore the incredible features and functionalities of React.js, a powerful JavaScript library for building user interfaces.
Specifically, we will delve into the world of React Hooks, a game-changing addition to React.js that will revolutionize the way you develop web applications.
React Hooks
React Hooks are a set of functions introduced in React 16.8 that enable developers to add stateful logic and other React features to functional components. Before the advent of Hooks, complex logic and state management in React components were primarily achieved using class components. However, Hooks provides a more streamlined and concise approach to working with state, side effects, and other React features within functional components.
Hooks were introduced to address several challenges associated with class components, such as code reuse, state management, and lifecycle complexities. They allow developers to encapsulate logic into reusable functions, making components easier to read, test, and maintain. Hooks also promotes a more modular approach to building components, enhancing their reusability and composability.
The Power of React Hooks
React Hooks are the cool perks they bring to the table in our development journey. They’re like the superheroes of efficiency and maintainability in our applications. Ready to dive into some of the fantastic advantages React Hooks have in store for us?
1. Enhanced Code Reusability
- With React Hooks, you can easily encapsulate and reuse logic across different components. This promotes code reusability and reduces the amount of boilerplate code in your application.
- By creating custom hooks, you can extract common functionality and share it across multiple components, making your codebase more modular and maintainable.
2. Streamlined State Management
- State management is a crucial aspect of any web application. React Hooks simplifies state management by providing the
useState
hook, which allows functional components to hold and update their own state. - With
useState
, you no longer need to convert functional components into class components just for state management. This streamlined approach enhances code readability and improves the overall development experience.
3. Efficient Side Effect Handling
- Side effects, such as data fetching, subscriptions, or manually manipulating the DOM, are an integral part of many applications. React Hooks provide the
useEffect
hook, which allows you to perform side effects in functional components. - The
useEffect
hook ensures proper management of side effects throughout the component’s lifecycle, preventing memory leaks and other common issues.
4. Simplified Context Handling
- Context provides a way to share data between components without explicitly passing props at every level.
- React Hooks introduces the
useContext
hook, which allows functional components to access the context of a parent component directly. This eliminates the need for prop drilling, making your code cleaner and more efficient.
5. Improved Performance Optimization
- React Hooks enable efficient performance optimization through hooks like useCallback and useMemo.
- The
useCallback
hook memoizes callback functions, preventing unnecessary re-renders of child components. - Similarly, the
useMemo
hook memoizes the result of a computation, avoiding redundant calculations on each render. - These hooks enhance the performance of your React application, particularly in scenarios where expensive calculations or frequent re-renders occur.
6. Simplified Testing
- Testing is an essential part of the development process.
- React Hooks makes testing easier by allowing you to test individual hooks independently.
- Since hooks are just functions, you can write focused unit tests to ensure their correctness. This modular approach to testing improves the overall quality of your application and simplifies the debugging process.
7. Seamless Migration
- If you are already working with class components in your React project, don’t worry! React Hooks provides a seamless migration path.
- You can gradually refactor your codebase and start using hooks alongside class components.
- This incremental approach allows you to leverage the benefits of hooks without having to rewrite your entire application.
Exploring Essential React Hooks
Now that we understand the power and benefits of React Hooks, let’s explore some essential hooks that will supercharge your web development process. These hooks will enhance your productivity, improve code quality, and unlock new possibilities in your React applications.
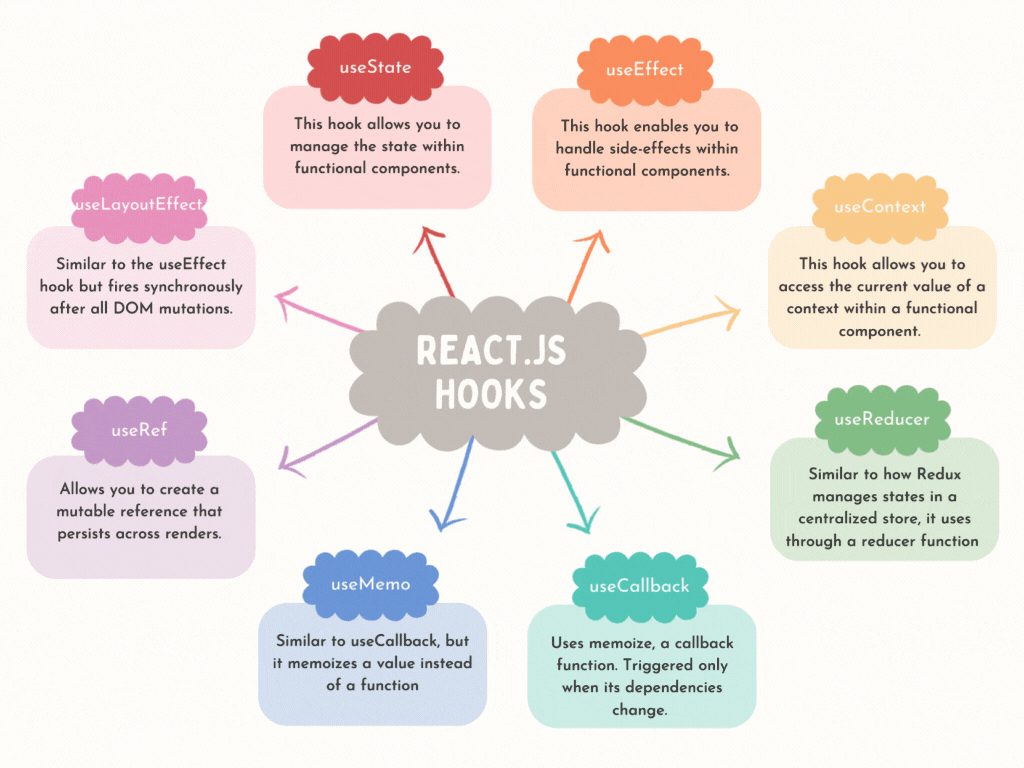
1. useState: Managing State in Functional Components
The useState hook is the cornerstone of React Hooks. It allows functional components to hold and update their own state, eliminating the need for class components. With useState
, you can easily manage the state within your functional components, making your code more concise and readable.
To use the useState
hook, you need to import it from the ‘react’ package. Let’s take a look at an example:
import { useState } from "react"; export default function Counter() { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; return ( <div> <h2>A simple click counter!</h2> <div> <p>Counter: {count}</p> <button onClick={increment}>Click me!</button> </div> </div> ); }
In this example, we define a Counter component that uses the useState hook to manage the count state. Initially, the count is set to 0, and each time the increment button is clicked, the count is updated using the setCount function.
2. useEffect: Handling Side Effects
The useEffect
hook allows you to perform side effects in functional components. Side effects are actions that don’t directly relate to rendering, such as data fetching, subscriptions, or DOM manipulation. The useEffect
hook ensures that these side effects are properly managed throughout the component’s lifecycle.
To use the useEffect
hook, you need to import it from the ‘react’ package. Let’s see an example of using useEffect
to fetch data from an API:
import { useState, useEffect } from "react"; export default function FetcData() { const [data, setData] = useState(null); useEffect(() => { const fetchData = async () => { const response = await fetch( "https://jsonplaceholder.typicode.com/todos" ); const jsonData = await response.json(); setData(jsonData); }; fetchData(); }, []); return ( <div> <h1>Todo's:</h1> {data ? ( <ul> {data.map((item) => ( <li key={item.id}> [{item.id}] {item.title} -{" "} <label>Status: {item.completed ? "yes" : "no"}</label> </li> ))} </ul> ) : ( <p>Loading data...</p> )} </div> ); }
In this example, the DataFetcher component uses the useEffect hook to fetch data from an external API. The useEffect
hook ensures that the data is fetched only once, thanks to the empty dependency array as the second argument. Any changes to the dependency array would trigger the effect to run again.
3. useContext: Accessing Context Easily
Context provides a way to share data between components without passing props at every level. The useContext hook allows functional components to access the context of a parent component directly, eliminating the need for prop drilling.
To use the useContext hook, you need to import it from the ‘react’ package. Let’s consider an example where we have a ThemeContext to manage the theme of our application:
import React, { createContext, useContext, useState } from 'react'; const ThemeContext = React.createContext('light'); const ThemeProvider = ({ children }) => { const [theme, setTheme] = useState('light'); const toggleTheme = () => { setTheme((prevTheme) => (prevTheme === 'light' ? 'dark' : 'light')); }; return ( <ThemeContext.Provider value={{ theme, toggleTheme }}> {children} </ThemeContext.Provider> ); }; const ThemedComponent = () => { // Access the context using useContext const { theme, toggleTheme } = useContext(ThemeContext); return ( <div style={{ background: theme === 'light' ? '#fff' : '#333', color: theme === 'light' ? '#333' : '#fff', padding: '20px', textAlign: 'center' }}> <h2>Themed Component</h2> <p>Current Theme: {theme}</p> <button onClick={toggleTheme}>Toggle Theme</button> </div> ); }; export default function App() { return ( <ThemeProvider> <div> <h2>My App - Theme Managment</h2> <ThemedComponent /> </div> </ThemeProvider> ) }
In this example, the ThemeComponent component uses the useContext hook to access the theme value from the ThemeContext. Depending on the theme value, the button’s background and text color will be set accordingly.
4. useReducer: Managing Complex State Transitions
The useReducer
hook provides an alternative way to manage complex state logic within functional components. It is often used as an alternative or complement to the useState
hook, especially when state updates involve intricate logic or transitions between multiple states.
To use the useReducer
hook, you need to import it from the ‘react’ package. Let’s consider an example where we use useReducer to manage a simple counter:
import React, { useReducer } from "react"; // Initial state const initialState = { count: 0 }; // Reducer function const reducer = (state, action) => { switch (action.type) { case "increment": return { count: state.count + 1 }; case "decrement": return { count: state.count - 1 }; case "reset": return { count: 0 }; default: throw new Error("Unknown action type"); } }; // Component const Counter = () => { const [state, dispatch] = useReducer(reducer, initialState); const handleIncrement = () => { dispatch({ type: "increment" }); }; const handleDecrement = () => { dispatch({ type: "decrement" }); }; const handleReset = () => { dispatch({ type: "reset" }); }; return ( <div> <h1>Simple Counter that increments, decrements & resets counter!</h1> <h2>Counter: {state.count}</h2> <button onClick={handleIncrement}>Increment +1</button> <button onClick={handleDecrement}>Decrement -1</button> <button onClick={handleReset}>Reset Counter</button> </div> ); }; export default Counter;
In this example, we define a Counter component that uses the useReducer hook to manage the count state. The reducer function handles the state transitions based on the dispatched actions. By using the dispatch function, we can update the state according to the action type.
5. useRef: Accessing DOM Elements
The useRef
hook provides a way to create a mutable reference to a value that persists across component renders. It is often used for accessing DOM elements, holding onto values that don’t trigger re-renders, and other scenarios where you need to maintain a reference to something that’s not part of the component’s state.
To use the useRef
hook, you need to import it from the ‘react’ package. Let’s consider an example where we use useRef to focus on an input element:
import { useRef, useEffect } from "react"; export default function Form() { const inputRef = useRef(); // Reference Object useEffect(() => { inputRef.current.focus(); inputRef.current.placeholder = "enter your text here!"; }, []); return ( <> <h2>Sample code using useRef!</h2> <input ref={inputRef} type="text" />; </> ); }
In this example, the InputFocus component uses the useRef hook to create a reference to the input element. When the “Focus Input” button is clicked, the focusInput function is called, which uses the ref to focus the input element.
6. useCallback: Memoizing Callback Functions
The useCallback
hook is used to optimize the performance of functional components by memoizing and reusing callback functions. It is particularly useful when dealing with scenarios where components are frequently re-rendered due to changes in their props or state.
To use the useCallback
hook, you need to import it from the ‘react’ package. Let’s consider an example where we use useCallback
to optimize the rendering of a child component:
import React, { useCallback, useState } from "react"; export default function Counter() { const [count, setCount] = useState(0); const increment = useCallback(() => { setCount((prevCount) => prevCount + 1); }, []); return ( <div> <h2>A simple click counter!</h2> <p>Counter: {count}</p> <button onClick={increment}>Increment +1</button> <p>{count && count === 5 ? "Give me high five 🫸...!" : ""}</p> </div> ); }
In this example, the ParentComponent uses the useCallback hook to memoize the handleClick function. This ensures that the handleClick function is not recreated on every render unless the count value changes.
7. useMemo: Memoizing Expensive Computations
The useMemo
hook is used to optimize performance by memoizing the result of a computation. It is especially useful when dealing with expensive calculations or when you want to avoid re-computing a value on every render of a functional component.
To use the useMemo
hook, you need to import it from the ‘react’ package. Let’s consider an example where we use useMemo to avoid redundant calculations:
import React, { useMemo, useState } from "react"; const SampleComponent = () => { const [inputValue, setInputValue] = useState(""); const memoizedValue = useMemo(() => { return complexCalculation(inputValue); }, [inputValue]); const handleInputChange = (e) => { setInputValue(e.target.value); }; return ( <div> <h1>Enter your favourite text and see it repeat ~ infinity!</h1> <input type="text" required value={inputValue} onChange={handleInputChange} /> <p> <b>Result:</b> <br /> {memoizedValue} </p> </div> ); }; // A complex calculation function const complexCalculation = (input) => { // Perform some time-consuming calculations let result = ""; // Example of a time-consuming operation for (let i = 0; i < 10000; i++) { result += input; } return result; }; export default SampleComponent;
In this example, the Sample component uses the useMemo hook to memoize the result value. The expensive computation is performed only when the data prop changes, preventing unnecessary recalculations on subsequent renders.
8. Custom Hooks: Encapsulating Logic
Custom hooks allow you to encapsulate and reuse logic in your components, making your code cleaner, more maintainable, and easier to test. By creating custom hooks, you can extract common functionality and share it across multiple components, promoting code reusability and separation of concerns.
To create a custom hook, you simply define a JavaScript function that starts with the word “use” and leverages built-in hooks or other custom hooks. Let’s consider an example of a custom hook that provides a convenient way to work with the browser’s local storage:
import { useState } from 'react'; const UseLocalStorage = (key, initialValue) => { const [storedValue, setStoredValue] = useState(() => { try { const item = window.localStorage.getItem(key); return item ? JSON.parse(item) : initialValue; } catch (error) { console.error(error); return initialValue; } }); const setValue = (value) => { try { const valueToStore = value instanceof Function ? value(storedValue) : value; setStoredValue(valueToStore); window.localStorage.setItem(key, JSON.stringify(valueToStore)); } catch (error) { console.error(error); } }; return [storedValue, setValue]; }; const MyComponent = () => { const [value, setValue] = UseLocalStorage('myKey', 'defaultValue'); // Rest of your component logic using value and setValue return ( <div> <h2>LocalStorage - Reusable Compoenent</h2> <p>Local Stored Value: {value}</p> <button onClick={() => setValue('Updated Value')}>Update Value</button> </div> ); }; export default MyComponent;
In this example, the useLocalStorage custom hook provides a convenient way to work with the browser’s local storage. It returns a value and a setter function that can be used to store and retrieve data from the local storage. The initial value is retrieved from the local storage, and any subsequent updates are also persisted.
Conclusion
Congratulations! You have embarked on a journey into the fascinating world of React Hooks and discovered their immense power in supercharging your web development projects. With the ability to enhance code reusability, streamline state management, handle side effects efficiently, and simplify testing, React Hooks revolutionize the way you build React applications.
We explored essential hooks like useState, useEffect, useContext, useReducer, useRef, useCallback, and useMemo, along with the notion of custom hooks. These hooks empower you to build highly efficient, scalable, and maintainable applications, while also unleashing your creativity and productivity as a web developer.
As you continue your React.js journey, don’t forget to leverage the power of React Hooks and explore their potential in various real-world use cases. Whether you’re building form validations, implementing authentication and authorization, handling real-time data updates, or venturing into mobile development with React Native, React Hooks will be your trusty companions.
So, go forth and embrace the power of React Hooks in your web development projects.
Happy coding, fellow developers! 🚀